Socket Io Document
First we need to bind socket.io to our HTTP server. That would be the first line of code - piece of cake. The next block is the reason we're all here - by calling io.set('authorization', function (handshakeData, accept) we instruct socket.io to run our authorization code when performing a handshake. SocketIO for Unity - v1.0.0 - # Overview # This plugin allows you to integrate your Unity game with Socket.IO back-end It implements the protocol described at socket.io-protocol github repo.
A minimalistic, single-file client implementation. Since socket-io is a dying technology, and the specification is quite complicated, bugs on either side might just never get fixed at any time. A single file approach is at least easier to tune, expand and debug. A practice to movement of 3D characters based on a network system. (Unity 3D, Node JS, Socket IO) Throughout these repository, I’ll make practice about how to create my multiplayer game with a dedicated server driven by NodeJs. This will be built on top of websockets so that it is responsive allowing for real-time multiplayer game play. Server side prediction with socket.io and Unity 3d In this tutorial I will explain how to prevent speed hacking in a node js server, with Unity as the client. If you are familiarized with multiplayer game development, you’ve probably heard of “client side prediction”.
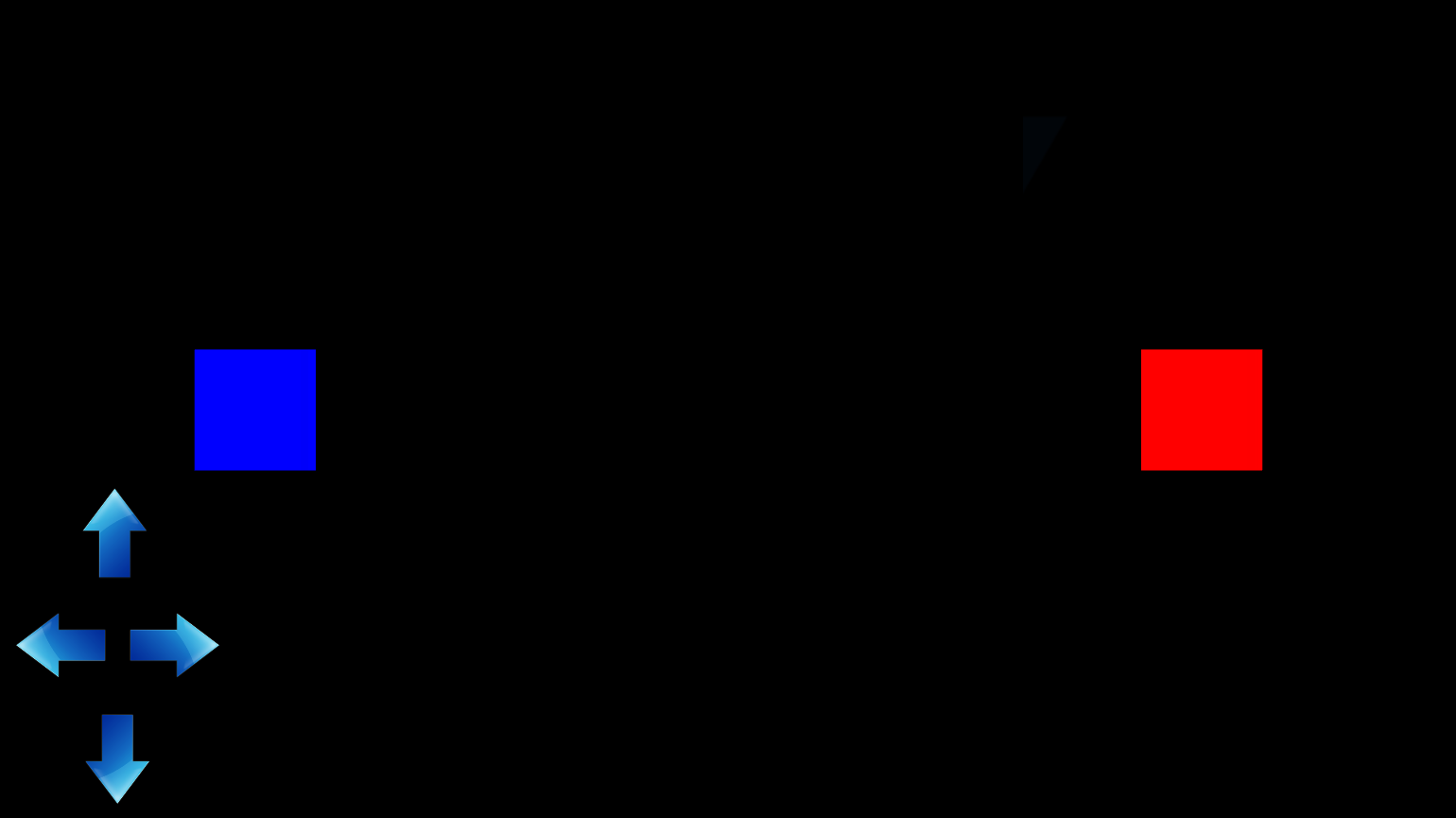
In this guide we’ll create a basic chat application. It requires almost no basic prior knowledge of Node.JS or Socket.IO, so it’s ideal for users of all knowledge levels.
Introduction
Writing a chat application with popular web applications stacks like LAMP (PHP) has normally been very hard. It involves polling the server for changes, keeping track of timestamps, and it’s a lot slower than it should be.
Sockets have traditionally been the solution around which most real-time chat systems are architected, providing a bi-directional communication channel between a client and a server.
This means that the server can push messages to clients. Whenever you write a chat message, the idea is that the server will get it and push it to all other connected clients.
The web framework
The first goal is to set up a simple HTML webpage that serves out a form and a list of messages. We’re going to use the Node.JS web framework express
to this end. Make sure Node.JS is installed.
First let’s create a package.json
manifest file that describes our project. I recommend you place it in a dedicated empty directory (I’ll call mine chat-example
).
Now, in order to easily populate the dependencies
property with the things we need, we’ll use npm install
:
Once it’s installed we can create an index.js
file that will set up our application.
This means that it:
- Express initializes
app
to be a function handler that you can supply to an HTTP server (as seen in line 4). - We define a route handler
/
that gets called when we hit our website home. - We make the http server listen on port 3000.
If you run node index.js
you should see the following:
And if you point your browser to http://localhost:3000
:
Serving HTML
So far in index.js
we’re calling res.send
and passing it a string of HTML. Our code would look very confusing if we just placed our entire application’s HTML there, so instead we’re going to create a index.html
file and serve that instead.
Let’s refactor our route handler to use sendFile
instead.
Put the following in your index.html
file:
If you restart the process (by hitting Control+C and running node index.js
again) and refresh the page it should look like this:
Integrating Socket.IO
Socket.IO is composed of two parts:
- A server that integrates with (or mounts on) the Node.JS HTTP Server socket.io
- A client library that loads on the browser side socket.io-client
Unity3d Socket Io
During development, socket.io
serves the client automatically for us, as we’ll see, so for now we only have to install one module:
That will install the module and add the dependency to package.json
. Now let’s edit index.js
to add it:
Notice that I initialize a new instance of socket.io
by passing the server
(the HTTP server) object. Then I listen on the connection
event for incoming sockets and log it to the console.
Now in index.html add the following snippet before the </body>
(end body tag):
That’s all it takes to load the socket.io-client
, which exposes an io
global (and the endpoint GET /socket.io/socket.io.js
), and then connect.
If you would like to use the local version of the client-side JS file, you can find it at node_modules/socket.io/client-dist/socket.io.js
.
Notice that I’m not specifying any URL when I call io()
, since it defaults to trying to connect to the host that serves the page.
If you now restart the process (by hitting Control+C and running node index.js
again) and then refresh the webpage you should see the console print “a user connected”.
Try opening several tabs, and you’ll see several messages.
Each socket also fires a special disconnect
event:
Then if you refresh a tab several times you can see it in action.
Emitting events
The main idea behind Socket.IO is that you can send and receive any events you want, with any data you want. Any objects that can be encoded as JSON will do, and binary data is supported too.
Let’s make it so that when the user types in a message, the server gets it as a chat message
event. The script
section in index.html
should now look as follows:
And in index.js
we print out the chat message
event:
The result should be like the following video:
Broadcasting
The next goal is for us to emit the event from the server to the rest of the users.
In order to send an event to everyone, Socket.IO gives us the io.emit()
method.
If you want to send a message to everyone except for a certain emitting socket, we have the broadcast
flag for emitting from that socket:
In this case, for the sake of simplicity we’ll send the message to everyone, including the sender.
And on the client side when we capture a chat message
event we’ll include it in the page. The total client-side JavaScript code now amounts to:
And that completes our chat application, in about 20 lines of code! This is what it looks like:
Homework
Here are some ideas to improve the application:
- Broadcast a message to connected users when someone connects or disconnects.
- Add support for nicknames.
- Don’t send the same message to the user that sent it. Instead, append the message directly as soon as he/she presses enter.
- Add “{user} is typing” functionality.
- Show who’s online.
- Add private messaging.
- Share your improvements!
Socket Io Unity Client
Getting this example
You can find it on GitHub here.
Like many homes, my kids and I enjoy playing video games together. At this stage of life, my kids especially playing games like Minecraft or Roblox. As I continue to explore the Unity ecosystem, I decided to look into using NodeJs to implement a playground for my kids in a Unity game. In this post, I’ll outline the major ideas I used to create my prototype. In the future, hope this prototype evolves into a multiplayer tank shooter game.
This blog post will assume a working knowledge of Unity. To start things off, I installed the following tools from the Unity Asset store. If you’re interested in getting started with Unity, check out their learning materials here.
https://assetstore.unity.com/packages/tools/network/socket-io-for-unity-21721(by Fabio Panettieri)
https://assetstore.unity.com/packages/tools/input-management/json-net-for-unity-11347
(from Parent Element LLC)
The SocketIO asset includes a sample scene outlining the common setup.
If you’re interested in inspecting a finished sample, please visit the following GitHub link.
https://github.com/michaelprosario/tanks
I did test this SocketIO in an Android mobile environment too. Unfortunately, it didn’t work well. These patterns seem to work fine with Unity desktop experiences. There may be additional edits I need to do to get it working.
While learning this pairing of Unity 3D and SocketIO, I found the following video series very helpful too. Thank you Adam Carnagey!
To build our prototype, let’s kick things off by building our server. This server will leverage NodeJs. In your server working directory, create a package.json like the following:
Next, let’s implement our tank-server.js. This code will instantiate a server using web sockets at port 4567.
Implementing the Unity client controller
In your Unity solution, you will go through a process of creating a scene and defining your environment. In this section, we’ll outline the major parts of implementing the socket io prefab and the controller script.
- Like any game experience, we start with creating a new unity project, adding a scene, making a terrain, and player objects.
Install the Unity SocketIO package from the asset store. https://assetstore.unity.com/packages/tools/network/socket-io-for-unity-21721
After the package installs, inspect the README.txt and sample scene to get a feel of how you layout your scene and configure the references to your server. I recommend that you layout your scene in a similar fashion.
For my solution, I started by crafting a few command and query objects to communicate state changes of my players to the server. We’ll also review response objects to receive data from the server.
In this interface, we model some of the key operations of our scene. In your implementation of the scene, you will need to provide concrete implementations of these operations. You can see my simple implementation here:
https://github.com/michaelprosario/tanks/blob/master/Assets/Scenes/SceneOneView.cs
At this point, we’re ready to talk through the main socket controller script. I’m only going to highlight the key themes of the script. You can inspect the whole body of the script here. https://github.com/michaelprosario/tanks/blob/master/Assets/Scenes/SceneOneSocketController.cs
This controller depends upon the socketIO and a reference to the current player. The controller keeps a reference to the view interface so that messages from the server can be routed to scene actions.
When we start the controller, we give our player an identity. Next, we resolve the references for the view and the socket IO component. Finally, we connect various socket events to handlers. We also let the server know that a new player started.
The following methods enable us to send commands and query requests to the server.
To announce a new player, we leverage the following method.
As we move around, we let the server know how we’re moving. My gut says that I need to find a more optimal way to communicate this kind of state. I think it will work fine for a small number of players on a local network.
The following handlers map responses from the socket server to view actions.
Hope this post gives you a general overview of how to start a multi-player Unity scene using NodeJS and SocketIO. We love to hear from our readers. Please share a comment and let us know what you’re building!
